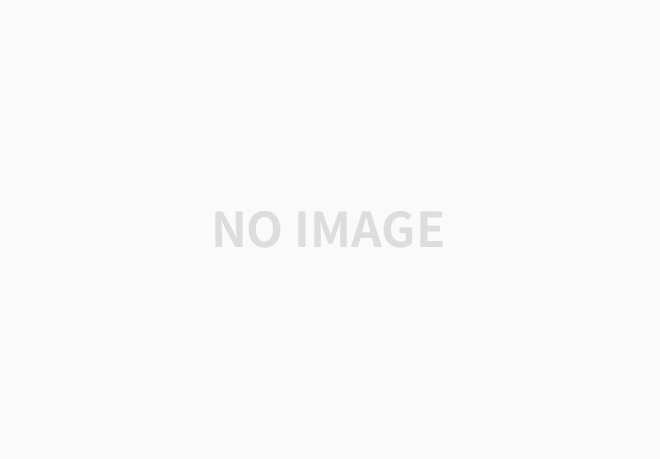
๋ชฉ์ฐจ
1. Stack
2. Stack ์ฌ์ฉํ๊ธฐ
- push()
- pop()
- remove()
- peek()
- isEmpty()
- empty()
- search()
- size()
- set()
- elementAt
- clear()
1. Stack (์คํ)
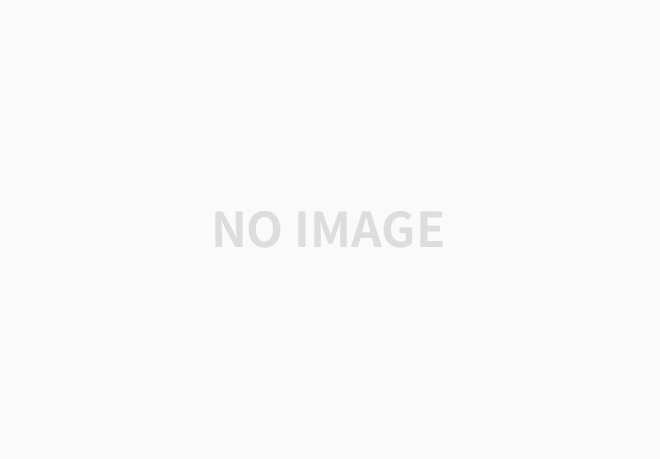
- ์คํ์ ๋ง์ง๋ง์ ๋ฃ์ ๋ฐ์ดํฐ๋ฅผ ๊ฐ์ฅ ๋จผ์ ๊บผ๋ธ๋ค. (ํ์ ์ ์ถ. LIFO)
* ์ฐธ๊ณ ๋ก Queue(ํ)๋ ์ ์ ์ ์ถ(FIFO)
- ์๋ฐ ๊ฐ์ ๋จธ์ (JVM)์ ์ค์ค๋ก ๋ฉ๋ชจ๋ฆฌ๋ฅผ ๊ด๋ฆฌํ๋ ๊ธฐ๋ฅ์ ํ๋๋ฐ,
์ด๋ Stack์ ๋ง์ง๋ง์ผ๋ก ์ฌ์ฉ์ด ๋๋ ์ง์ญ๋ณ์๋ฅผ ๋ฐ๋ก ์ณ๋ด๋ฒ๋ฆฌ๋ฏ๋ก, ๋งค์ฐ ํจ์จ์ ์ผ๋ก ๋ฉ๋ชจ๋ฆฌ๋ฅผ ์ฌ์ฉํ๋ ๋ฐฉ์์ด๋ค.
๋, ์๋ฐ์ Stack ํด๋์ค๋ Vector ํด๋์ค๋ฅผ ์์๋ฐ๋๋ค! ๋ฐ๋ผ์ Thread-safe ํน์ง์ ๊ฐ๋๋ค๊ณ ํ๋ค.
2. Stack ์ฌ์ฉํ๊ธฐ
๋จผ์ ์๋ฐ์์ Stack์ ์ฌ์ฉํ๊ธฐ ์ํด์ ,
import.java.util.Stack ์ ์ถ๊ฐํด์ค์ผ ํ๋ค.
์ ์ธ
Stack<์๋ฃํ> ๋ณ์๋ช = new Stack();
ใด ํด๋น ์๋ฃํ๋ง ์ฝ์ , ์ญ์ ๊ฐ๋ฅํ๋ค.
Stack ๋ณ์๋ช = new Stack();
ใด์ด๋ค ์๋ฃํ์ด๋ ์ฝ์ , ์ญ์ ๊ฐ๋ฅ(int ํ๊ณผ Stringํ ํผํฉ ์ฝ์ ๊ฐ๋ฅ)
2-1. ๋ฉ์๋ ์ ๋ฆฌ
push() - ์ฝ์
import java.util.Stack;
public class StackPractice1 {
public static void main(String[] args) {
Stack<Number> stack = new Stack<>();
stack.push(1);
stack.push(2);
stack.push(3);
System.out.println(stack); //[1, 2, 3]
}
}
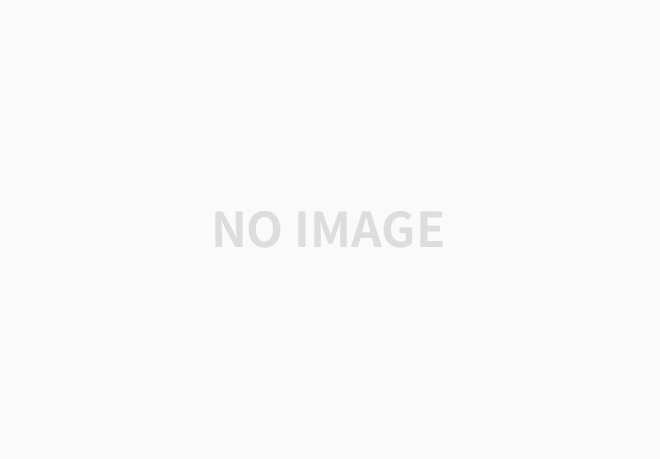
pop( ) - ์ญ์
System.out.println(stack); //[1, 2, 3]
System.out.println(stack.pop()); //3
System.out.println(stack); //[1, 2]
System.out.println(stack.pop()); //2
pop()์ ํ๋ฉด ์คํ์ ๊ฐ์ฅ ์๋ถ๋ถ, ์ฆ ๋ง์ง๋ง์ push()ํด์ค ๊ฐ๋ถํฐ ๊บผ๋ด์ง๋ค.
์ stack์ ๊ฒฝ์ฐ, 3->2->1 ์.
remove( index ) - ์ญ์
ํน์ ์ธ๋ฑ์ค๊ฐ ๊ฐ๋ฆฌํค๋ ๊ฐ์ ์ญ์ ํ ๋
Stack<String> stack = new Stack();
stack.push("Hello");
stack.push("goodbye");
stack.push("zoo");
stack.push("banana");
stack.push("monkey");
stack.remove(3);
System.out.println(stack); //[Hello, goodbye, zoo, monkey]
ใด ์ธ๋ฑ์ค 3์ด ๊ฐ๋ฆฌํค๋ banana๊ฐ ์ญ์ ๋จ.
peek( ) - ๊ฐ์ฅ ์์ ์์๊ฐ
import java.util.Stack;
public class StackPractice1 {
public static void main(String[] args) {
Stack<Number> stack = new Stack<>();
stack.push(1);
stack.push(2);
stack.push(3);
System.out.println(stack.peek()); //3
}
}
isEmpty( ) - ์คํ์ด ๋น์ด์๋?
empty( ) - ์คํ์ด ๋น์ด์๋?
์คํ์ pop ํ๊ฑฐ๋ peek ํ ๋,
์คํ ๋ด๋ถ์ ๋ฐ์ดํฐ๊ฐ ํ๋๋ ์๋ ์ํฉ์ด๋ผ๋ฉด, EmptyStackException ์์ธ๊ฐ ๋ฐ์ํ๋ค.
์ด๋ฅผ ๋ฐฉ์งํ๊ธฐ ์ํด isEmpty() ๋ฉ์๋๋ก ์คํ์ด ๋น์ด์๋์ง ํ์ธ ๊ฐ๋ฅํ๋ค.
-> boolean ๊ฐ์ผ๋ก ์ถ๋ ฅ๋๋ค.
-> if๋ฌธ์ ์กฐ๊ฑด์ผ๋ก ์ฌ์ฉํ์ฌ ์์ธ๋ฐ์ ๋ฐฉ์ง
Stack<Number> stack = new Stack<>();
stack.push(1);
stack.push(2);
stack.push(3);
System.out.println(stack.peek()); //3
System.out.println(stack.isEmpty()); //false
if(!stack.isEmpty()) {
stack.pop();
System.out.println(stack); //[1, 2]
}
search( ์ฐพ์ value๊ฐ ) - ํด๋น ์์๊ฐ ์๋๊ฐ?
์คํ ๋ด๋ถ์ ํน์ ๋ฐ์ดํฐ๊ฐ ์กด์ฌํ๋์ง ๊ฒ์, ํ์ธํ๋ ๋ฉ์๋.
*๋ฆฌ์คํธ์ indexOf() ๋ฉ์๋์ ๋น์ทํ๋ค.
๋ฐ์ดํฐ๊ฐ ์กด์ฌํ๋ฉด -> ๋ฐ์ดํฐ์ ์ธ๋ฑ์ค๋ฅผ ๋ฐํํจ.
์กด์ฌํ์ง ์์ผ๋ฉด -> -1 ๋ฐํํจ.
ใด๊ตณ์ด
Stack<String> stack = new Stack();
stack.push("Hello");
stack.push("goodbye");
stack.push("zoo");
stack.push("banana");
stack.push("monkey");
System.out.println(stack.search("zoo")); //3
System.out.println(stack.search("rabbit")); //-1
size( ) - ์คํ์ ํฌ๊ธฐ
์๋ฐ์ Stackํด๋์ค๋ Vectorํด๋์ค๋ฅผ ์์ํ๊ณ ,
Vector ํด๋์ค๋ List ์ธํฐํ์ด์ค๋ฅผ ๊ตฌํํ๊ธฐ ๋๋ฌธ์
Stack ํด๋์ค์์ ์คํ์ ํฌ๊ธฐ๋ฅผ ๋ํ๋ผ๋ size() ๋ฉ์๋๋ฅผ ์ฌ์ฉํ ์ ์๋ค.
Stack<String> stack = new Stack();
stack.push("Hello");
stack.push("goodbye");
stack.push("zoo");
stack.push("banana");
stack.push("monkey");
System.out.println(stack.size()); //5
set( index, ๋ณ๊ฒฝํ value ) - ์คํ ๊ฐ ๋ณ๊ฒฝ
Stack<String> stack = new Stack();
stack.push("Hello");
stack.push("goodbye");
stack.push("zoo");
stack.push("banana");
stack.push("monkey");
stack.set(3, "apple");
System.out.println(stack); //[Hello, goodbye, zoo, apple, monkey]
clear ( ) - ์คํ ์ด๊ธฐํ / ์คํ์ ๊ณต๋ฐฑ์ผ๋ก ๋ง๋ฆ.
: ๋น์์ค ๋ ์ฌ์ฉํจ.
*Stack ํด๋์ค๋ Vector ํด๋์ค์ ์ปฌ๋ ์ ์ ์์๋ฐ์ ๊ตฌํ๋๋๋ฐ,
vector ํด๋์ค๋ '์ปฌ๋ ์ ํ๋ ์์ํฌ'์ ๊ฐ๋ ์ด ๋์ค๊ธฐ๋ ์ ์ ์๋ฐ 1 ๋ฒ์ ๋ถํฐ ์๋ ์ค๋๋ ์ปฌ๋ ์ ์ด๊ธฐ ๋๋ฌธ์ ๋ถ์์ ํ๋ค.
์ด๋ฐ vector ํด๋์ค(์ฆ, ๋ถ์ ํฉํ ๋ถ๋ชจ ํด๋์ค)๋ฅผ ์์๋ฐ๋ Stack ํด๋์ค์ ์ธ์คํด์ค ์ํ๊ฐ ๋ถ์์ ํด์ง๋ค๋ ๋จ์ ์ด ์๋ค.
โก๏ธ vector ํด๋์ค : add()
โก๏ธ stack ํด๋์ค : push()
์ด๋ฐ ๋ถ์์ ํจ ๋๋ฌธ์ ์๋ฐ๋ ์ฐ๋ฆฌ์๊ฒ stack ๋์ Deque ํด๋์ค ์ฌ์ฉ์ ๊ถ์ฅํ๋ค.
Deque(๋ฑ) : ์๋ฐฉํฅ์ผ๋ก ๋ฃ๊ณ ๊บผ๋ผ ์ ์๋ ํ(Queue)์ด๋ค. -> ํ์ฒ๋ผ ์ฌ์ฉํ ์ ๋ ์๊ณ , stack์ฒ๋ผ ์ฌ์ฉํ ์ ๋ ์๋ค.
*๋ด์ฉ ์ฐธ๊ณ : Dev inpa TISTORY BLOG
'๐ป > JAVA' ์นดํ ๊ณ ๋ฆฌ์ ๋ค๋ฅธ ๊ธ
[JAVA] ๋ฒกํฐ(vector) ยท ๋ฉ์๋ ์ ๋ฆฌ (0) | 2024.10.14 |
---|---|
[JAVA] ํ(Queue) ยท ๋ฉ์๋ ์ ๋ฆฌ (0) | 2024.10.14 |
[JAVA] sortํจ์, ๋ฐฐ์ด ์ ๋ ฌ(์ค๋ฆ์ฐจ์, ๋ด๋ฆผ์ฐจ์) (0) | 2024.10.14 |
[JAVA] String ๋น๊ตํ๊ธฐ (5) | 2024.09.30 |
[JAVA] Java์ ์ธ์ ์ ๋ฌ , ํฌ์ธํฐ ? - call by value, call by reference (0) | 2024.09.29 |